1110. Delete Nodes And Return Forest
Description
Given the root
of a binary tree, each node in the tree has a distinct value.
After deleting all nodes with a value in to_delete
, we are left with a forest (a disjoint union of trees).
Return the roots of the trees in the remaining forest. You may return the result in any order.
Example 1:
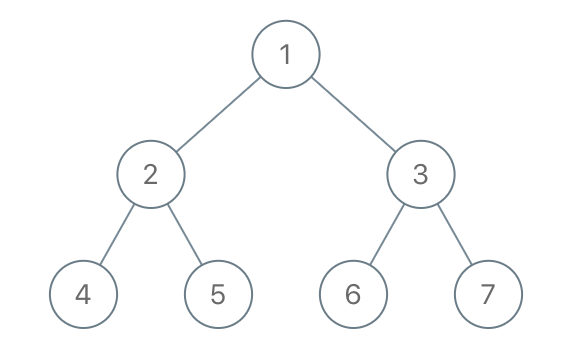
Input: root = [1,2,3,4,5,6,7], to_delete = [3,5] Output: [[1,2,null,4],[6],[7]]
Example 2:
Input: root = [1,2,4,null,3], to_delete = [3] Output: [[1,2,4]]
Constraints:
- The number of nodes in the given tree is at most
1000
. - Each node has a distinct value between
1
and1000
. to_delete.length <= 1000
to_delete
contains distinct values between1
and1000
.
Solutions
Solution: Depth-First Search
- Time complexity: O(n)
- Space complexity: O(n)
JavaScript
js
/**
* Definition for a binary tree node.
* function TreeNode(val, left, right) {
* this.val = (val===undefined ? 0 : val)
* this.left = (left===undefined ? null : left)
* this.right = (right===undefined ? null : right)
* }
*/
/**
* @param {TreeNode} root
* @param {number[]} to_delete
* @return {TreeNode[]}
*/
const delNodes = function (root, to_delete) {
const result = [];
const dfsTreeNode = node => {
if (!node) return null;
const { left, right, val } = node;
node.left = dfsTreeNode(left);
node.right = dfsTreeNode(right);
if (to_delete.includes(val)) {
node.left && result.push(left);
node.right && result.push(right);
return null;
}
return node;
};
if (!to_delete.includes(root.val)) result.push(root);
dfsTreeNode(root);
return result;
};