1074. Number of Submatrices That Sum to Target
Description
Given a matrix
and a target
, return the number of non-empty submatrices that sum to .
A submatrix x1, y1, x2, y2
is the set of all cells matrix[x][y]
with x1 <= x <= x2
and y1 <= y <= y2
.
Two submatrices (x1, y1, x2, y2)
and (x1', y1', x2', y2')
are different if they have some coordinate that is different: for example, if x1 != x1'
.
Example 1:
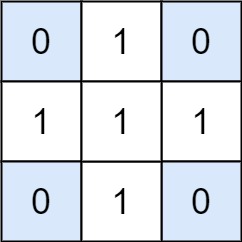
Input: matrix = [[0,1,0],[1,1,1],[0,1,0]], target = 0 Output: 4 Explanation: The four 1x1 submatrices that only contain 0.
Example 2:
Input: matrix = [[1,-1],[-1,1]], target = 0 Output: 5 Explanation: The two 1x2 submatrices, plus the two 2x1 submatrices, plus the 2x2 submatrix.
Example 3:
Input: matrix = [[904]], target = 0 Output: 0
Constraints:
1 <= matrix.length <= 100
1 <= matrix[0].length <= 100
-1000 <= matrix[i][j] <= 1000
-10^8 <= target <= 10^8
Solutions
Solution: Prefix Sum
- Time complexity: O(mn2)
- Space complexity: O(m)
JavaScript
js
/**
* @param {number[][]} matrix
* @param {number} target
* @return {number}
*/
const numSubmatrixSumTarget = function (matrix, target) {
const m = matrix.length;
const n = matrix[0].length;
let result = 0;
for (let row = 0; row < m; row++) {
for (let col = 1; col < n; col++) {
matrix[row][col] += matrix[row][col - 1];
}
}
for (let left = 0; left < n; left++) {
for (let right = left; right < n; right++) {
const sumCountMap = new Map([[0, 1]]);
let sum = 0;
for (let row = 0; row < m; row++) {
sum += matrix[row][right];
if (left > 0) sum -= matrix[row][left - 1];
result += sumCountMap.get(sum - target) ?? 0;
sumCountMap.set(sum, (sumCountMap.get(sum) ?? 0) + 1);
}
}
}
return result;
};