1721. Swapping Nodes in a Linked List
Description
You are given the head
of a linked list, and an integer k
.
Return the head of the linked list after swapping the values of the kth
node from the beginning and the kth
node from the end (the list is 1-indexed).
Example 1:
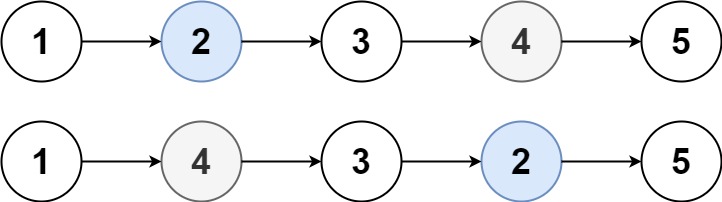
Input: head = [1,2,3,4,5], k = 2 Output: [1,4,3,2,5]
Example 2:
Input: head = [7,9,6,6,7,8,3,0,9,5], k = 5 Output: [7,9,6,6,8,7,3,0,9,5]
Constraints:
- The number of nodes in the list is
n
. 1 <= k <= n <= 105
0 <= Node.val <= 100
Solutions
Solution: Two Pointers
- Time complexity: O(n)
- Space complexity: O(1)
JavaScript
js
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} head
* @param {number} k
* @return {ListNode}
*/
const swapNodes = function (head, k) {
let current = (rightNode = head);
let leftNode = null;
let size = (index = 0);
while (current) {
size += 1;
if (size === k) leftNode = current;
current = current.next;
}
while (index < size - k) {
rightNode = rightNode.next;
index += 1;
}
[leftNode.val, rightNode.val] = [rightNode.val, leftNode.val];
return head;
};