335. Self Crossing
Description
You are given an array of integers distance
.
You start at the point (0, 0)
on an X-Y plane, and you move distance[0]
meters to the north, then distance[1]
meters to the west, distance[2]
meters to the south, distance[3]
meters to the east, and so on. In other words, after each move, your direction changes counter-clockwise.
Return true
if your path crosses itself or false
if it does not.
Example 1:
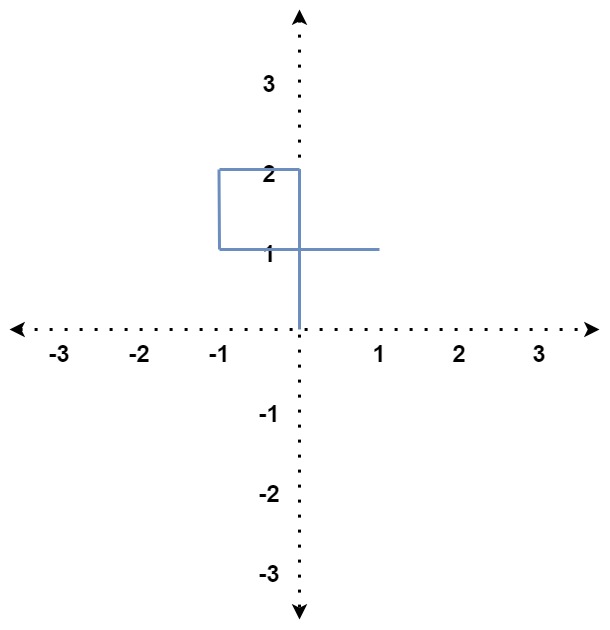
Input: distance = [2,1,1,2] Output: true Explanation: The path crosses itself at the point (0, 1).
Example 2:
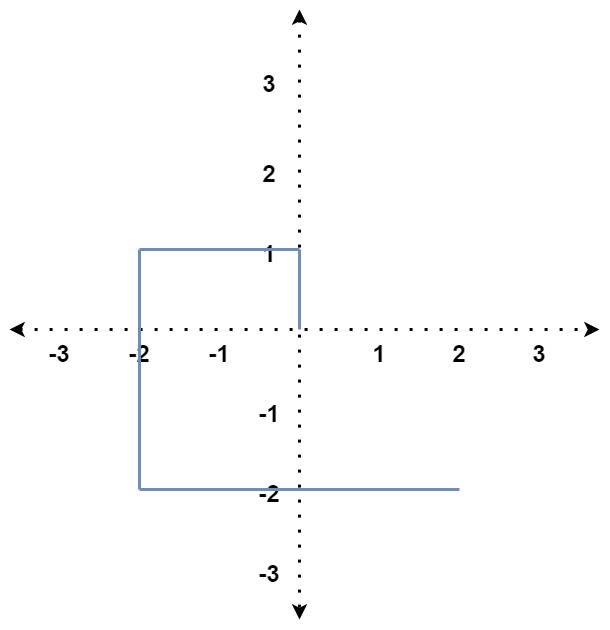
Input: distance = [1,2,3,4] Output: false Explanation: The path does not cross itself at any point.
Example 3:
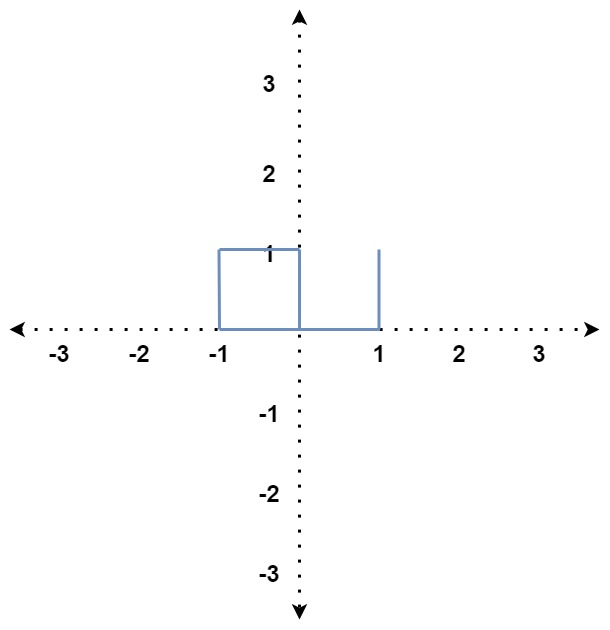
Input: distance = [1,1,1,2,1] Output: true Explanation: The path crosses itself at the point (0, 0).
Constraints:
1 <= distance.length <= 105
1 <= distance[i] <= 105
Solutions
Solution: Math
- Time complexity: O(n)
- Space complexity: O(1)
JavaScript
js
/**
* @param {number[]} distance
* @return {boolean}
*/
const isSelfCrossing = function (distance) {
const situation1 = index => {
const one = distance[index - 3];
const two = distance[index - 2];
const three = distance[index - 1];
const four = distance[index];
return one >= three && four >= two;
};
const situation2 = index => {
if (index < 4) return false;
const one = distance[index - 4];
const two = distance[index - 3];
const three = distance[index - 2];
const four = distance[index - 1];
const five = distance[index];
return three >= one && two === four && five >= three - one;
};
const situation3 = index => {
if (index < 5) return false;
const one = distance[index - 5];
const two = distance[index - 4];
const three = distance[index - 3];
const four = distance[index - 2];
const five = distance[index - 1];
const six = distance[index];
return four >= two && three >= five && one >= three - five && six >= four - two;
};
for (let index = 3; index < distance.length; index++) {
if (situation1(index)) return true;
if (situation2(index)) return true;
if (situation3(index)) return true;
}
return false;
};