1584. Min Cost to Connect All Points
Description
You are given an array points
representing integer coordinates of some points on a 2D-plane, where points[i] = [xi, yi]
.
The cost of connecting two points [xi, yi]
and [xj, yj]
is the manhattan distance between them: |xi - xj| + |yi - yj|
, where |val|
denotes the absolute value of val
.
Return the minimum cost to make all points connected. All points are connected if there is exactly one simple path between any two points.
Example 1:
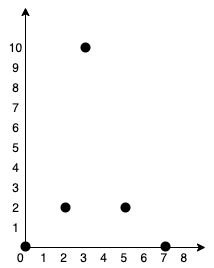
Input: points = [[0,0],[2,2],[3,10],[5,2],[7,0]] Output: 20 Explanation:We can connect the points as shown above to get the minimum cost of 20. Notice that there is a unique path between every pair of points.
Example 2:
Input: points = [[3,12],[-2,5],[-4,1]] Output: 18
Constraints:
1 <= points.length <= 1000
-106 <= xi, yi <= 106
- All pairs
(xi, yi)
are distinct.
Solutions
Solution: Minimum Spanning Tree
- Time complexity: O(n2logn)
- Space complexity: O(n2)
JavaScript
js
/**
* @param {number[][]} points
* @return {number}
*/
const minCostConnectPoints = function (points) {
const size = points.length;
const graph = new Array(size)
.fill('')
.map((_, index) => index);
const costs = [];
for (let a = 0; a < size - 1; a++) {
for (let b = a + 1; b < size; b++) {
const [x1, y1] = points[a];
const [x2, y2] = points[b];
const cost = Math.abs(x1 - x2) + Math.abs(y1 - y2);
costs.push({ cost, a, b });
}
}
costs.sort((a, b) => a.cost - b.cost);
return costs.reduce((result, { cost, a, b }) => {
const rootA = unionFind(a);
const rootB = unionFind(b);
if (rootA === rootB) return result;
graph[rootA] = graph[rootB];
return result + cost;
}, 0);
function unionFind(target) {
if (graph[target] === target) return target;
graph[target] = unionFind(graph[target]);
return graph[target];
}
};